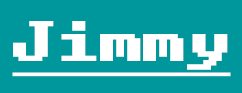
import React, { Component } from 'react'; import { AppRegistry, Dimensions,StyleSheet, Text,TextInput, View ,Button,FlatList,Image,DeviceEventEmitter} from 'react-native'; const styles = require('../Resource/defaultStyle/ControlScreenStyle'); import DetailScreen from './DetailScreen'; import mqtt from '../util/mqtt'; var connectedObj=null; class ControlScreen extends React.Component { constructor(props) { super(props); this.state = { backData: [] }; //this.state = {text: '',address:'ws://39.106.111.41:8885',user:'mosquitto',pass:'',message:'',subTitle:'/jimmy',pubTitle:'/jimmy',pubContent:''}; this.state = {text: '',address:'wss://wq.jimmycarbin.com',user:'mosquitto',pass:'',message:'',subTitle:'/jimmy',pubTitle:'/jimmy',pubContent:''}; }; // static navigationOptions = { // title: 'Xenstat', // }; static navigationOptions = { header: null, }; randomString(len) { len = len || 32; var $chars = 'ABCDEFGHJKMNPQRSTWXYZabcdefhijkmnprstwxyz2345678'; /****默认去掉了容易混淆的字符oOLl,9gq,Vv,Uu,I1****/ var maxPos = $chars.length; var pwd = ''; for (var i = 0; i < len; i++) { pwd += $chars.charAt(Math.floor(Math.random() * maxPos)); } return pwd; } componentDidMount() { } componentWillMount(){ } componentDidUpdate() { } componentWillUnmount() { } connectMqtt(){ var that = this; if(!this.state.address){ return false; } that.client = mqtt.connect(that.state.address,{ clientId: that.randomString(12), clean:true, username: that.state.user, password: that.state.pass, reconnectperiod:3000 }); console.log(that.client); that.client.on('connect', function() { console.warn('connected'); //client.subscribe('/jimmy', 0); //client.publish('/jimmy', "test", 0, false); that.connectedObj = that.client; that.setState({message:'服务器连接成功'}); }); that.client.on('closed', function() { console.log('mqtt.event.closed'); }); that.client.on('error', function(msg) { console.warn('mqtt.event.error', msg); }); that.client.on('message', function(topic,msg) { console.warn('mqtt.event.message', msg); //var newMsg = JSON.Parse(msg); //console.warn('mqtt.event.message', newMsg.data); that.setState({message:"接收到 "+topic+" 的消息:"+msg.toString()}); }); } issueSub(){ var that = this; if(that.connectedObj == null|| typeof that.connectedObj == undefined ||typeof that.connectedObj.options == undefined){ that.setState({message:'请先链接服务器'}); return false; } if(!that.state.subTitle){ that.setState({message:'请输入订阅主题'}); return false; } that.connectedObj.subscribe(that.state.subTitle, 0); that.setState({message:'订阅主题'+that.state.subTitle+'成功!'}); } issuePub(){ var that = this; if(that.connectedObj == null|| typeof that.connectedObj == undefined ||typeof that.connectedObj.options == undefined){ that.setState({message:'请先链接服务器'}); return false; } if(!that.state.pubTitle||!that.state.pubContent){ that.setState({message:'请输入发布主题或内容'}); return false; } that.connectedObj.publish(that.state.pubTitle, that.state.pubContent, 0, false); that.setState({message:'向主题'+that.state.pubTitle+' 发布'+that.state.pubContent +'成功!'}); } render() { const { navigate } = this.props.navigation; var that = this; return ( <View style={styles.outerContainer}> {/* <View style={styles.inputBox}> <TextInput style={styles.inputSty} placeholder="Type here to search!" underlineColorAndroid='white' onChangeText={(text) => this.setState({text})} /> <Text style={styles.searchSty}>搜索</Text> </View> <View style={styles.topLine}></View> */} <View style={styles.inputBox}> <Text style={styles.searchSty}>地址:</Text> <TextInput style={styles.inputSty} placeholder={this.state.address?this.state.address:'请输入服务器地址:mqtt://host:port'} underlineColorAndroid='white' onChangeText={(address) => this.setState({address})} /> </View> <View style={styles.topLine}></View> <View style={styles.inputBox}> <Text style={styles.searchSty}>用户:</Text> <TextInput style={styles.inputSty} placeholder={this.state.user?this.state.user:'请输入用户:'} underlineColorAndroid='white' onChangeText={(user) => this.setState({user})} /> </View> <View style={styles.topLine}></View> <View style={styles.inputBox}> <Text style={styles.searchSty}>密码:</Text> <TextInput style={styles.inputSty} placeholder={this.state.pass?this.state.pass:'请输入密码:'} underlineColorAndroid='white' onChangeText={(pass) => this.setState({pass})} /> </View> <View style={styles.topLine}></View> <Button title={'连接服务器'} onPress={() => this.connectMqtt() } /> <View style={styles.inputBox}> <Text style={styles.searchSty}>订阅主题:</Text> <TextInput style={styles.inputSty} placeholder={this.state.subTitle?this.state.subTitle:'请输入订阅主题:'} underlineColorAndroid='white' onChangeText={(subTitle) => this.setState({subTitle})} /> </View> <Button title={'订阅'} onPress={() => this.issueSub() } /> <View style={styles.topLine}></View> <View style={styles.inputBox}> <Text style={styles.searchSty}>发布主题:</Text> <TextInput style={styles.inputSty} placeholder={this.state.pubTitle?this.state.pubTitle:'请输入发布主题:'} underlineColorAndroid='white' onChangeText={(pubTitle) => this.setState({pubTitle})} /> </View> <View style={styles.inputBox}> <Text style={styles.searchSty}>发布内容:</Text> <TextInput style={styles.inputSty} placeholder={this.state.pubContent?this.state.pubContent:'请输入发布内容:'} underlineColorAndroid='white' onChangeText={(pubContent) => this.setState({pubContent})} /> </View> <Button title={'发布'} onPress={() => this.issuePub() } /> <View style={styles.topLine}></View> <Text> 收到的消息:{this.state.message}</Text> </View> ); } } export default ControlScreen