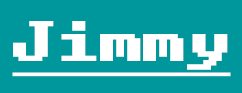
/** * 首屏白屏解决方法: * https://github.com/crazycodeboy/react-native-splash-screen/blob/master/README.zh.md * * * NetInfo https://reactnative.cn/docs/0.42/netinfo.html * **/ import React, {Component} from 'react'; import { View, Text, TextInput, Image, DeviceEventEmitter, TouchableOpacity, Alert, StatusBar, Platform, WebView, Keyboard, TouchableWithoutFeedback, NetInfo } from 'react-native'; // import {isFirstTime,markSuccess} from 'react-native-update'; import {NavigationActions} from 'react-navigation'; //获取服务器地址 const Url='http://ip.shishicai456.com/url.json'; // const Url='http://192.168.10.13:666/url.json'; //获取任务链接地址 const Url_task='http://appupdate.shishicai456.com/taskConfig.json'; //const Url_task='http://192.168.10.13:666/taskConfig.json'; const styles = require('../Resource/defaultStyle/LoginPageStyle'); const images = require('../Resource/defaultImg/LoginPageImg'); var sendParams = require('../WsSupport/sendParams'); var owner = require('../Business/UserInfo/getOwner'); var session = require('../Business/UserInfo/getSession'); var Global = require('../WsSupport/connecting'); var localstore = require('../base/aboutLocalStorage/localStore'); var connectSocketServer = require('../WsSupport/webConnection'); import Orientation from 'react-native-orientation'; import KeyboardSpacer from 'react-native-keyboard-spacer'; import DropdownAlert from 'react-native-dropdownalert'; import home from './MainPage'; import modifyPassWord from './modifyPassWord/modifyPassWord'; import SplashScreen from 'react-native-splash-screen' import commonFun from '../base/commonFun/commonFun' import LoadingModal from './modal/loadingModalPage'; class LoginPage extends React.Component { static navigationOptions = { header: null, }; constructor(props) { super(props); this.state = { user: '', pwd: '', }; Global.showTask=true; this.isRelogin = false; Global.roadInfo=new Array(); this.isConneted = true; } async getUrl(){ try { DeviceEventEmitter.emit("loading", true); let response = await fetch(Url); Global.roadData = await response.text(); connectSocketServer.dropalert=this._dropdown; connectSocketServer.getSpeed(); //DeviceEventEmitter.emit("loading", false); } catch (ex){ DeviceEventEmitter.emit("loading", false); } } getTaskUrl() { fetch(Url_task) .then((res)=>res.json()) .then((data)=>Global.isShowTask = data.showTask == 1) } componentDidMount() { SplashScreen.hide(); NetInfo.isConnected.addEventListener( 'connectionChange', this.handleFirstConnectivityChange.bind(this) ); NetInfo.isConnected.fetch().done( (isConnected) => this.handleFirstConnectivityChange(isConnected) ); this.getUrl(); this.getTaskUrl(); this.reLogin(); } componentWillMount(){ // if(isFirstTime){ // markSuccess(); // } Orientation.lockToPortrait(); } componentDidUpdate() { } componentWillUnmount() { this.reLoginListerner.remove(); NetInfo.isConnected.removeEventListener( 'connectionChange', this.handleFirstConnectivityChange.bind(this) ); } handleFirstConnectivityChange(isConnected) { //alert(isConnected); if(isConnected && !this.isConneted) { //alert('重连了!'); this.getUrl(); this.getTaskUrl(); connectSocketServer.getSpeed(); } this.isConneted = isConnected; } reLogin() { var methodName = "Relogin"; this.reLoginListerner = DeviceEventEmitter.addListener(methodName, (jsonObj, error)=> { var data = jsonObj; if (data == null || data.length == 0) { var Msg = "登入失败,请重试"; this._dropdown.alertWithType('error', '错误', Msg); //Alert.alert(Msg); return; } Global.Owner = owner.Owner(); Global.SessionID = data; owner.setOwner(Global.Owner); session.setSession(data); this.isRelogin = true; const {dispatch} = this.props.navigation; const resetAction = NavigationActions.reset({ index: 0, actions: [ NavigationActions.navigate({routeName: 'Main'}), ] }); dispatch(resetAction); }); } loginClick() { //const {navigate} = this.props.navigation; //navigate('Main',{name: 'home'}); // if(!connectSocketServer.connectState){ // this.getUrl(); // this.getTaskUrl(); // connectSocketServer.getSpeed(); // //console.warn('网络连接中请耐心等待!请稍后再试!'); // this._dropdown.alertWithType('error','错误','网络连接中请耐心等待!请稍后再试!'); // return; // } if (this.state.user == '' || this.state.pwd == '') { this._dropdown.alertWithType('error', '错误', '用户名或密码不能为空'); //Alert.alert('用户名或密码不能为空'); return; } var methodName = "Login"; this.listerner = DeviceEventEmitter.addListener(methodName, (jsonObj, error) => { DeviceEventEmitter.emit("loading", false); try { this.listerner.remove(); const {navigate} = this.props.navigation; if (error[0]) { var errorMsg = error[1]; this._dropdown.alertWithType('error', '错误', errorMsg); /*如果登入报错,提示用户信息*/ //Alert.alert(errorMsg); } else { var data = jsonObj; if (data == null || data.length == 0) { var Msg = "登入失败,请重试"; this._dropdown.alertWithType('error','错误',Msg); //Alert.alert(Msg); return; } connectSocketServer.navigator = this.props.navigation.dispatch; Global.Owner = this.state.user; Global.SessionID = data.SessionID; owner.setOwner(this.state.user); session.setSession(data.SessionID); const {dispatch} = this.props.navigation; const resetAction = NavigationActions.reset({ index: 0, actions: [ NavigationActions.navigate({routeName: 'Main'}), ] }); dispatch(resetAction); //navigate('Main',{name: 'home'}) } } catch (ex) { } }); DeviceEventEmitter.emit("loading", true); var params = [this.state.user, this.state.pwd, Platform.OS == 'ios' ? '5' : '4']; sendParams.send(methodName, params); } findPassWord() { this.props.navigation.navigate('ModifyPassWord',{name:'忘记密码'}); } clearText(ref, type){ ref.clear(); if(type == 'user') { this.setState({user:''}); }else{ this.setState({pwd:''}); } } goToKefuWeb(){ let kefuUrl="https://v88.live800.com/live800/chatClient/chatbox.jsp?companyID=616403&configID=2593&jid=8610714831&s=1&info="; this.props.navigation.navigate('Kefu',{kefuUrl:kefuUrl}); } render() { return ( <View style={styles.container}> {/*<Image source={images.bgImg} >*/} <StatusBar hidden={true} translucent={true}/> {/*RN中WebView加载本地html页面,会把页面当成资源文件,而不会当成可执行代码。 需要把页面手动添加到Android或Ios目录下,然后引入打包后的文件路径!*/} {commonFun.isIphoneX() ? <Image style={styles.top_gradient} source={require('../Resource/images/loginPage/login_top_gradient.png')}/> : null} <WebView javaScriptEnabled={true} domStorageEnabled={true} onLoadStart={DeviceEventEmitter.emit('loading',true)} onLoadEnd={DeviceEventEmitter.emit('loading',false)} style={styles.bgView} source={{uri:Platform.OS==='android'?'file:///android_asset/parallaxDemo/index.html':'./parallaxDemo/index.html'}}> </WebView> {commonFun.isIphoneX() ? <Image style={styles.bottom_gradient} source={require('../Resource/images/loginPage/login_root_gradient.png')}/> : null} {/*遮罩, 禁止拖动*/} <TouchableWithoutFeedback onPressIn={()=>{Keyboard.dismiss()}}> <View style={styles.overLayout}/> </TouchableWithoutFeedback> <Image style={styles.style_logo_image} source={images.loginLogo}/> <View style={styles.textInputContainer}> <TextInput style={styles.style_user_input} placeholder='请输入账号' placeholderTextColor="#f2f2f2" numberOfLines={1} autoFocus={false} ref='userText' //returnKeyType="next" //iOS //returnKeyLabel="下一项" // android onChangeText={(text)=> this.setState({user: text})} underlineColorAndroid='transparent' textAlignVertival='center' textAlign='center' autoCapitalize={'none'} /> {this.state.user == '' ? null : <TouchableOpacity style={styles.delUserBtn} onPress={() => this.clearText(this.refs.userText,'user')} > <Image source={images.delBtn} style={styles.del} /> </TouchableOpacity>} <View style={styles.border}/> <TextInput style={styles.style_pwd_input} placeholder='请输入密码' placeholderTextColor="#f2f2f2" numberOfLines={1} ref='PswText' //returnKeyType="done" //iOS //returnKeyLabel="完成" // android onChangeText={(text)=>this.setState({pwd: text})} secureTextEntry={true} underlineColorAndroid='transparent' textAlignVertical='center' textAlign='center' /> {this.state.pwd == '' ? null : <TouchableOpacity style={styles.delPswBtn} onPress={() => this.clearText(this.refs.PswText,'pwd')} > <Image source={images.delBtn} style={styles.del} /> </TouchableOpacity>} <View style={styles.border}/> <KeyboardSpacer topSpacing={Platform.OS == 'ios' ? commonFun.picHeight(-400) : commonFun.picHeight(-450)} /> <TouchableOpacity activeOpacity={0.8} style={styles.style_view_button} onPress={this.loginClick.bind(this)}> <Image source={images.loginBtn} style={styles.loginBtnImg}> <Text style={styles.loginText} allowFontScaling={false}>登录</Text> </Image> </TouchableOpacity> </View> <View style={styles.loginBottom}> <TouchableOpacity onPress={this.findPassWord.bind(this)} style={styles.forgetPswBtn} > <Image source={images.forgetPsw} style={styles.forgetPswImg}/> <Text style={styles.style_bottom_text} allowFontScaling={false}>忘记密码</Text> </TouchableOpacity> <View style={styles.line}/> <TouchableOpacity style={styles.serviceBtn} onPress={this.goToKefuWeb.bind(this)} > <Image source={images.loginService} style={styles.style_service_image}/> <Text style={styles.style_bottom_text} allowFontScaling={false}>在线客服</Text> </TouchableOpacity> </View> <LoadingModal/> <View style={{position:'absolute',top:0,zIndex:999,width:commonFun.deviceWidth(),height:150}}> <DropdownAlert style={{zIndex:2}} ref={(ref) => this._dropdown = ref} updateStatusBar={false} translucent={true} /> </View> </View> ) } } export default LoginPage